The shell scripting is an linux based script which works on shell language. It uses Bash or sh as an interpreter to execute it. It is a basic command based script which can handle day-to-day tasks which require huge execution time. To learn basic shell scripting, you must have handson basic shell commands.
The shell script can make tasks simpler by simply automating them for day-to-day tasks like disk cleanup, purging policies, backups, log rotations, etc. The shell script itself can’t make automation like scheduling the tasks. We require a Linux utility known as cron jobs for scheduling tasks, which can execute scripts at a specified time for execution, which makes less human involvement.
Basic shell commands:
Read-only commands:
Files and directories management commands:
- cd: Change your current working directory.
- pwd: Print the absolute path of your current working directory.
- ls: List the contents (files and directories) of a directory.
- mkdir: Create a new directory.
- rmdir: Remove an empty directory.
- rm: Remove files or directories (use with caution).
- cp: Copy files or directories from one location to another.
- mv: Move or rename files or directories.
- cat: Display file content or concatenate files.
- head: Display the beginning lines of a file (default value is 10).
- tail: Display the ending lines of a file (default value is 10).
Text processing commands:
- grep: Search for lines matching a pattern in files.
- sed: Perform text transformations on an input stream.
- awk: Process text line by line, often extracting and manipulating fields.
- cut: Extract specific sections (bytes, characters, or fields) from lines.
- sort: Sort the lines of a text file or input.
- uniq: Report or filter out repeated adjacent lines in a file.
Note:
sed
can change file content. Double-check your command to protect your data.
Process management commands:
- ps: Shows a snapshot of running processes and their details.
- top: Provides a real-time, dynamic view of system processes and resource usage.
- pstree: Shows running processes in a hierarchical tree structure.
- ps_mem: Reports memory usage by program name (Note: Might need to install ps_mem).
Network management commands:
- nmap: Command used for network scanning for host discovery, port scanning, and service/OS detection.
- mtr: Provides a network diagnostic report showing the path, latency, and packet loss to a destination.
- traceroute: Traces and displays the route (hops) that network packets take to reach a destination.
- iftop: Shows a real-time view of network bandwidth usage on an interface, broken down by connection.
- ethtool: Allows viewing and modifying the parameters and statistics of network interface cards.
- ifconfig: (Legacy command) Configures and displays network interface network parameters like IP addresses.
- tcpdump: Captures and displays network packets that pass through a network interface based on filters.
Note: Most organizations avoid nmap because while scanning, it causes suspicious activity on the server.
Input/Output & Variable Commands:
echo
: A command to print text or variable values to standard output, usually adding a newline.printf
: A command to print formatted text or variable values to standard output using a format string.read
: A command to read a line of input from the user and store it in a variable.
Utility commands:
find
: finds real-time data in the system.locate
: locates system data from maintained database (updatedb command is required to up-to-date every day)xargs
: A command that reads items from standard input (like a list of files generated by find) and executes a specified command using those items as arguments, helping to handle long argument lists or run commands that don’t directly accept piped input.sleep
: holds the console for a specified time.date
: Shows the current date and time of the machine, can also show dates of the future or past as required (generally used for logging and log rotations via scripts).
Example Scripts:
Let’s see some example scripts that we can use in daily tasks to improve our productivity.
Script to check system health in a single click:
#!/bin/bash
# --- Script Name: health_check.sh ---
# A simple script to perform basic system health checks.
echo "--- System Health Check Report ---"
echo "Run Date: $(date)"
echo "----------------------------------"
echo
# 1. Check System Uptime and Load Average
echo "### System Uptime and Load Average ###"
uptime
echo "---"
echo
# 2. Check Disk Usage (Human-readable format)
echo "### Disk Usage ###"
df -h
echo "---"
echo
# 3. Check Memory Usage (Human-readable format)
echo "### Memory Usage ###"
free -h
echo "---"
echo
# 4. Check Top 5 Processes (by CPU usage - requires 'top' or 'htop')
# Using top: -b (batch mode), -n 1 (one iteration), -o %CPU (sort by CPU), head (first 7 lines: header + 5 processes)
if command -v top &> /dev/null; then
echo "### Top 5 CPU Consuming Processes ###"
top -bn1 -o %CPU | head -n 12
echo "---"
echo
else
echo "### Top Processes (requires 'top' command) ###"
echo "'top' command not found. Skipping process check."
echo "---"
echo
fi
# 5. Check Kernel Version
echo "### Kernel Version ###"
uname -a
echo "---"
echo
# 6. Check Logged-in Users
echo "### Logged-in Users ###"
who
echo "---"
echo
echo "--- Health Check Complete ---"
The expected output:
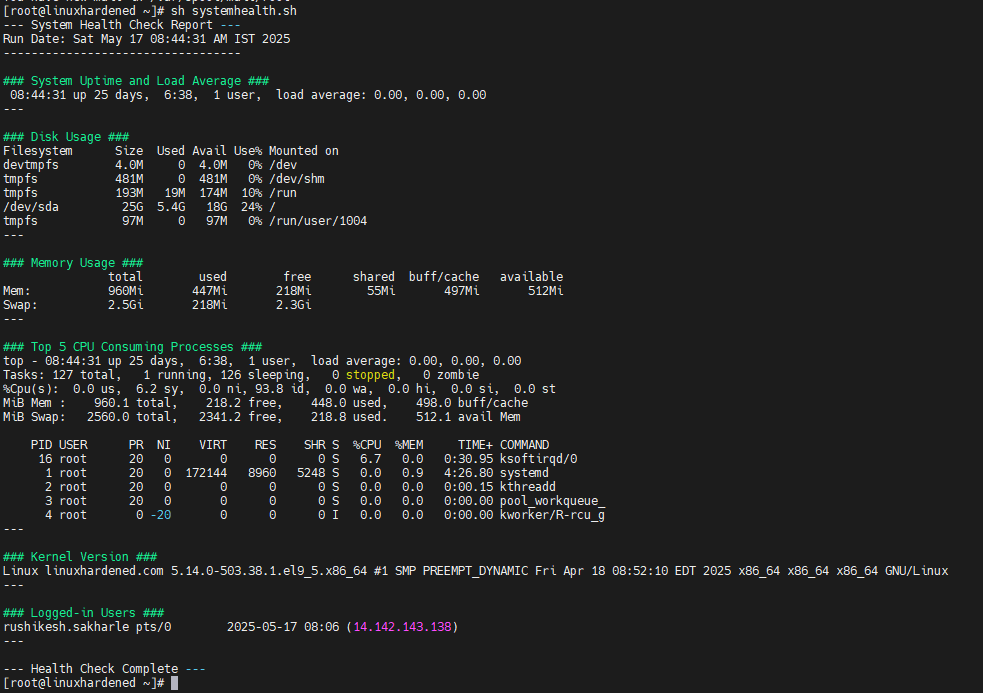
Conclusion:
Shell scripting automates Linux tasks using interpreters like Bash and basic commands. These commands cover essential areas like file management, text processing, and system monitoring. Scripts streamline repetitive day-to-day operations, improving efficiency. Task scheduling requires separate utilities like cron jobs, as scripts alone don’t handle it. Learning fundamental commands is key to writing effective scripts and understanding command arguments.